Controlling the display
Scrolling colors
Now we're going to use theĀ loop()Ā part of the code.
See thatĀ mp.update() function? It's probably the most important function of all and it's located under theĀ I/O section.
Its mission is to read everything that has happened between the last time it was called and nowĀ and to transfer those changes to the hardware.
For example, fillScreen() function would not change anything if the mp.update() isn't called.
Besides the screen, it also refreshes buttons, the speaker, LEDs and pretty much every other component on the phone.
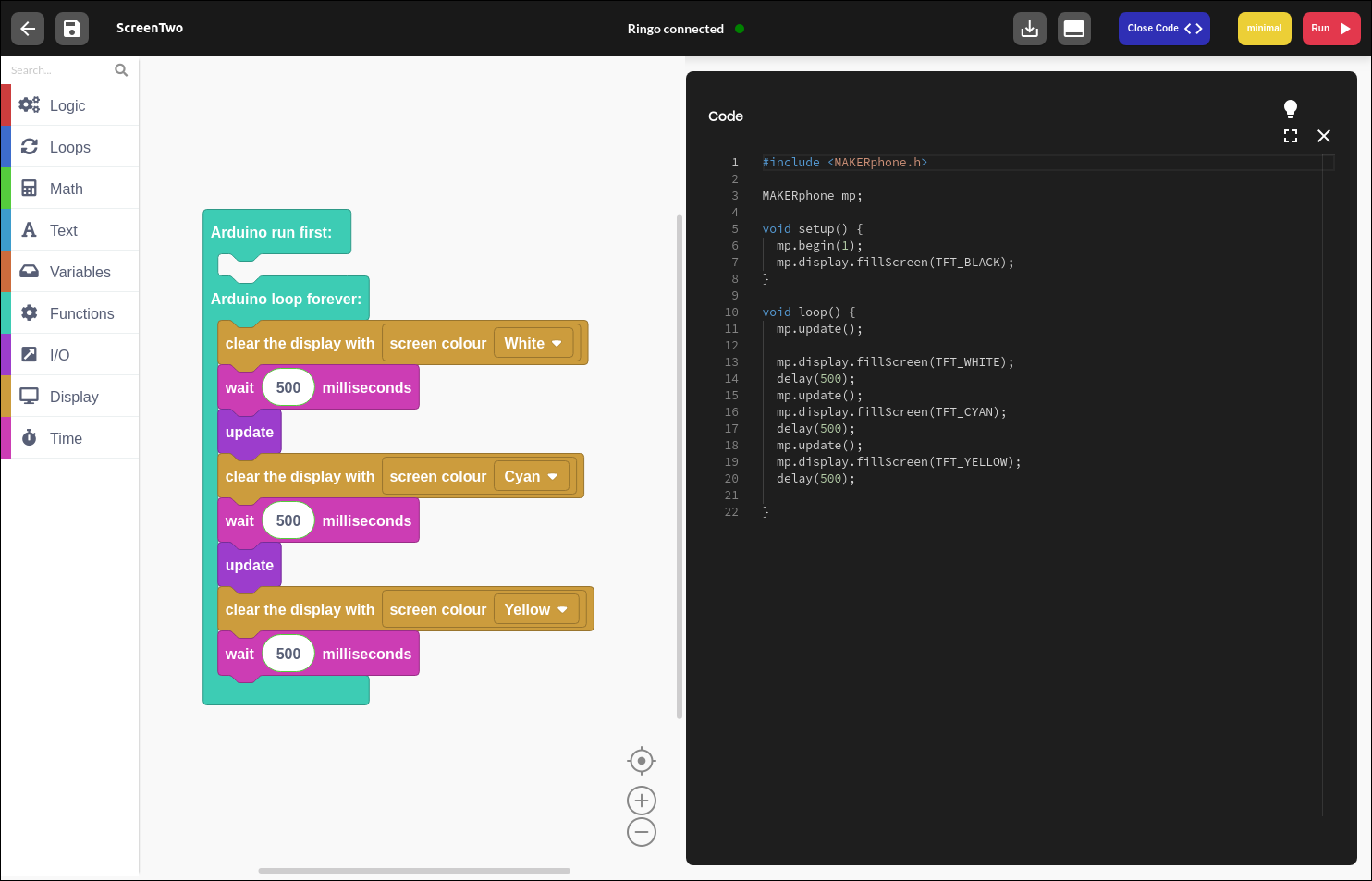
One mp.update() is there by default but for this program, we've added two more.
We've also used another new block calledĀ wait().
It translates to the delay() function which stops everything for a certain amount of milliseconds.
The number value block can be found in the Math section and its value can be changed to suit our needs.
Since the loop() function is really fast, sometimes we need this delay to actually see what is going on on the screen.
Using delay whenĀ expecting a fast and responsive program, however,Ā is not the way to go, especially when we're using buttons. More about that in the next lesson.
What we're actually doing in this program is alternating the screen color between three values.
Firstly we change it to white, then wait 500 milliseconds or 0.5 seconds.
Then, we change it to cyan and wait another 500 milliseconds.
Lastly, we change it to yellow, wait 500 milliseconds and return back to the beginning of the loop, where the screen is again changed to white.
Notice that we're calling the mp.update() function after each color change in order to transfer that color to the screen.
Update function
Be careful when using mp.update() since it is not the fastest function.Calling it too many times in one loop will slow down the program significantly - USE IT WELL!
Ringo library offers three different fonts that can be re-sized and re-colored as we like.
Functions for that are rather simple and should not be a problem to understand for anyone.
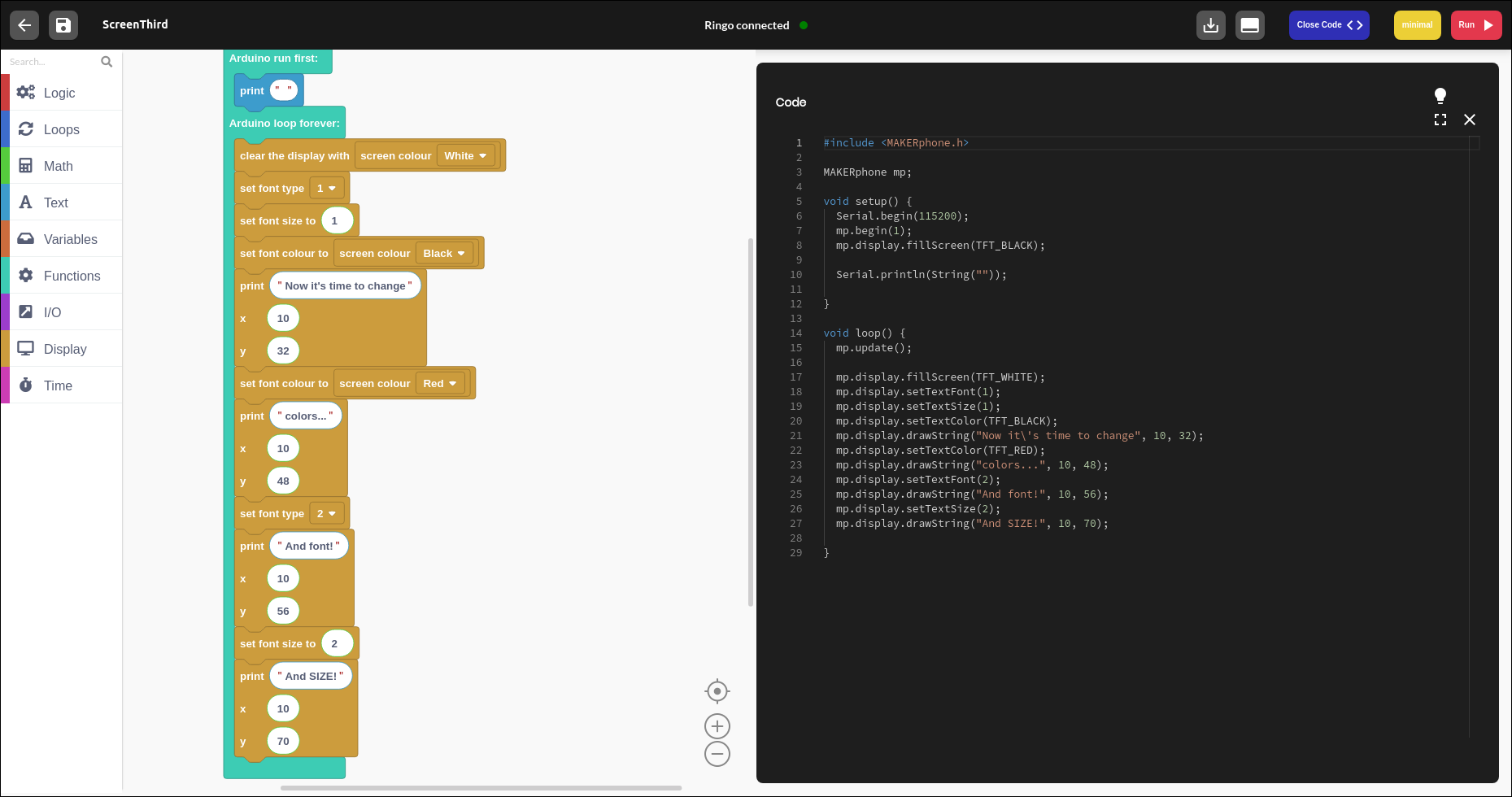
The font type is easily changed with theĀ drop-down menuĀ of the set font type block. The font size, however,Ā multiplies the size of the selected fontĀ by the desired value.Ā By default, both of those values are 1,Ā so setting them at the beginning of the program to that same value actually made no difference.
Later both of those values have been changed. In the last example, we're printing out two wordsĀ "And SIZE!"Ā in a different font that isĀ twice the size.
TheĀ print()Ā function here is something that we're going to use quite often. The first empty slot is a string of characters that are meant to be written out.
You can find an empty one in theĀ Text section.
The second and third slot are the location of the first letter.Ā Setting both of those values to 0 will print out words in the upper left corner of the screen.
With these numbers, we're actually referencingĀ pixel locations.Ā Our screen is 128x160 pixelsĀ and we can manipulate each and every one of them.
Everything that we write/draw between the x values of 0 and 127 and y values of 0 and 159 will be visible on the screen.
If any of those values is out of that range, we will not see the component.
Be careful when using mp.update() since it is not the fastest function.Calling it too many times in one loop will slow down the program significantly - USE IT WELL!
Writing out some text
Now that we know how to change the background color, it's time to start writing something on that canvas.
Ringo library offers three different fonts that can be re-sized and re-colored as we like.
Functions for that are rather simple and should not be a problem to understand for anyone.
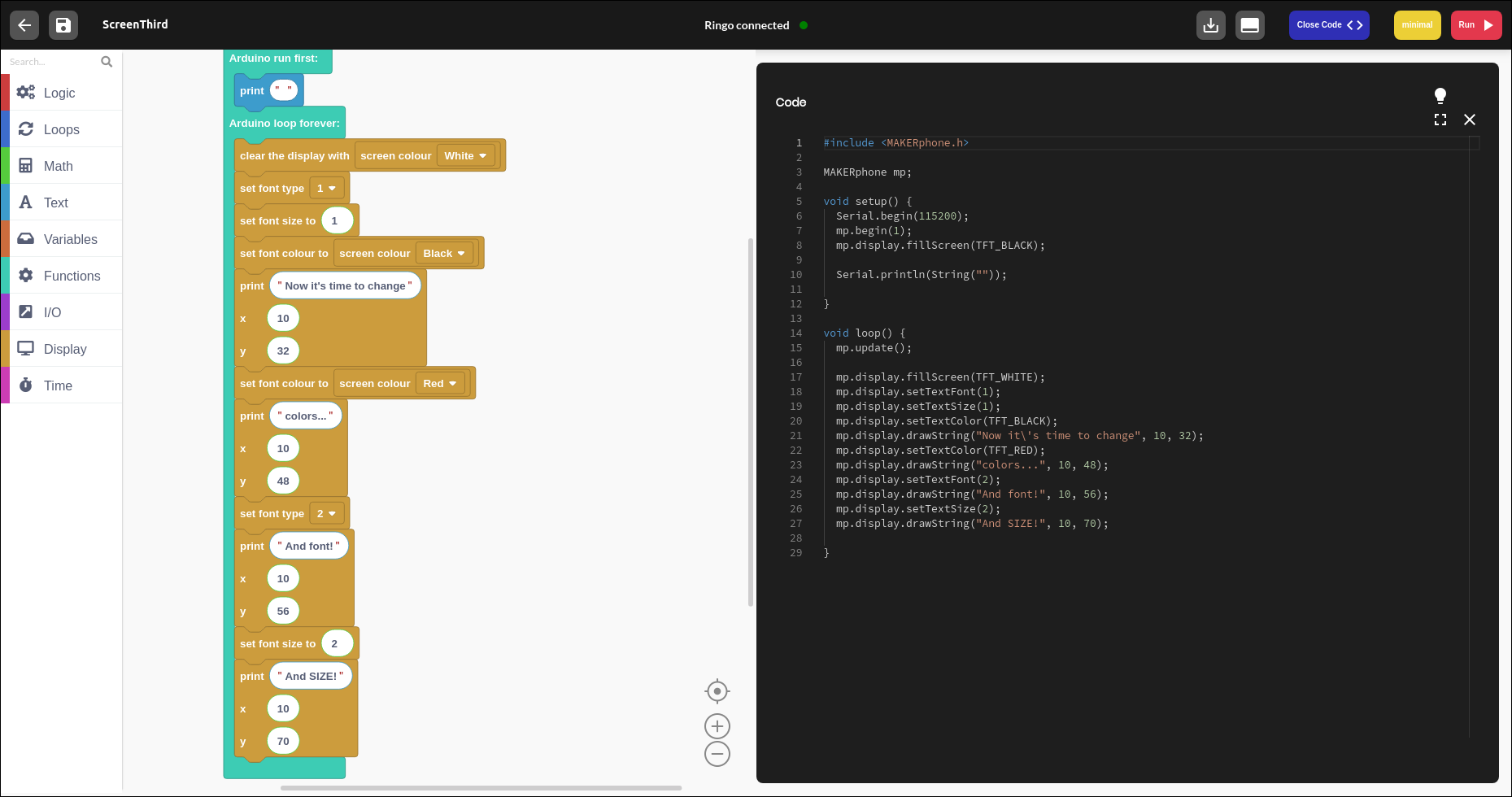
With this program, we're writing some words out on the screen inĀ different fonts, sizes, and colors.
The font type is easily changed with theĀ drop-down menuĀ of the set font type block. The font size, however,Ā multiplies the size of the selected fontĀ by the desired value.Ā By default, both of those values are 1,Ā so setting them at the beginning of the program to that same value actually made no difference.
Later both of those values have been changed. In the last example, we're printing out two wordsĀ "And SIZE!"Ā in a different font that isĀ twice the size.
TheĀ print()Ā function here is something that we're going to use quite often. The first empty slot is a string of characters that are meant to be written out.
You can find an empty one in theĀ Text section.
The second and third slot are the location of the first letter.Ā Setting both of those values to 0 will print out words in the upper left corner of the screen.
With these numbers, we're actually referencingĀ pixel locations.Ā Our screen is 128x160 pixelsĀ and we can manipulate each and every one of them.
Everything that we write/draw between the x values of 0 and 127 and y values of 0 and 159 will be visible on the screen.
If any of those values is out of that range, we will not see the component.
BackNext