Creating my fist app
Creating my fist app
Creating a whole app is not an easy task. There are a lot of little details that need to be considered in order to make the app work as good as possible.When creating your own app, always set the goals at the beginning, so you can work from the bottom up with some structure in mind.Writing code without a bigger picture in mind can be a big problem later on in the code development.
It will look like a simple video game, kind of like Snake, where you'll be able to move a circle and change its properties.
Main loop
This is what the main part of the program looks like. It doesn't say much since it has several functions that run the show in separate blocks.
You can notice the clever use of if-else here - a different function will be called depending on the value of the global variable color.
Using joystick
At the beginning of each loop, the program checks whether the joystick has been moved or not.
Placing multiple ifs instead of if-else we can get the diagonal movement, since one if doesn't exclude another.
The sensitivity of joystick can be changed by changing the values of comparison to the X and Y values.
LEDs
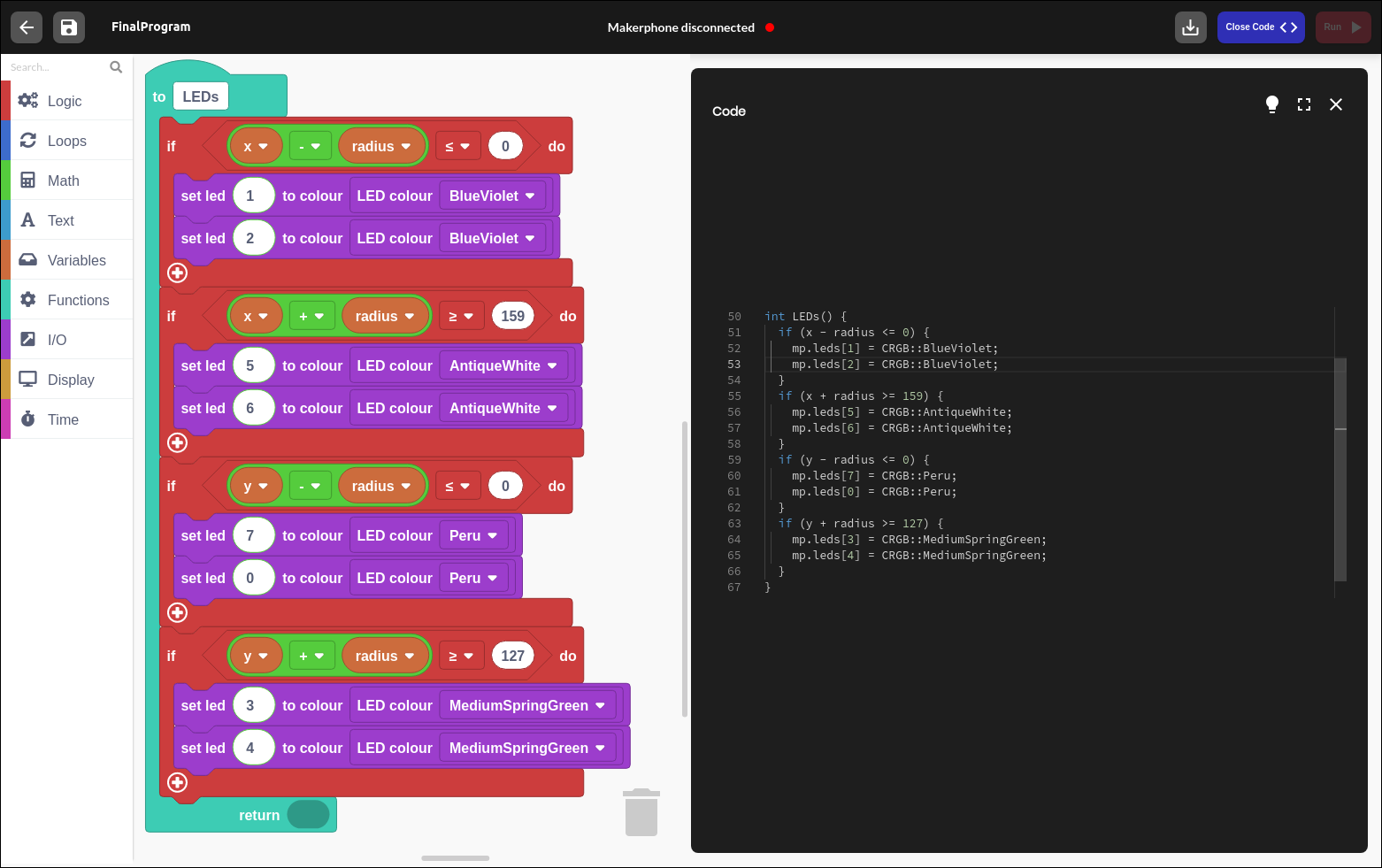
Notice that since both X and Y values are actually the center of the ball, we must keep in mind the radius size of our ball so that we know when the collision actually appears.
Since the length of the screen is 160 pixels and is taking up the values between 0 and 159, we are comparing it to the values of 0 and 159.
Same works with the height, where the most important values are 0 and 127.
Object size
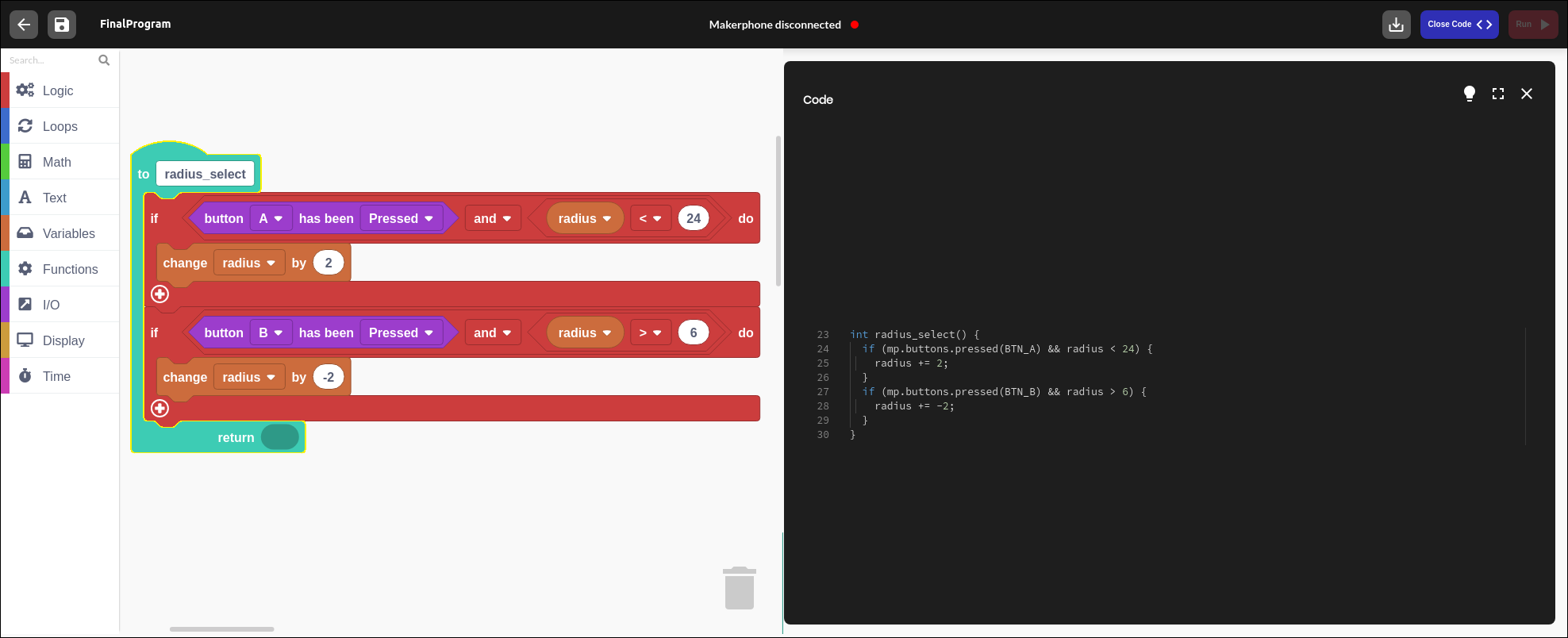
Button A will increase its size by two and button B will decrease it.
There are also checks at specific values so we can stop our circle from getting too big or too small.
Colors
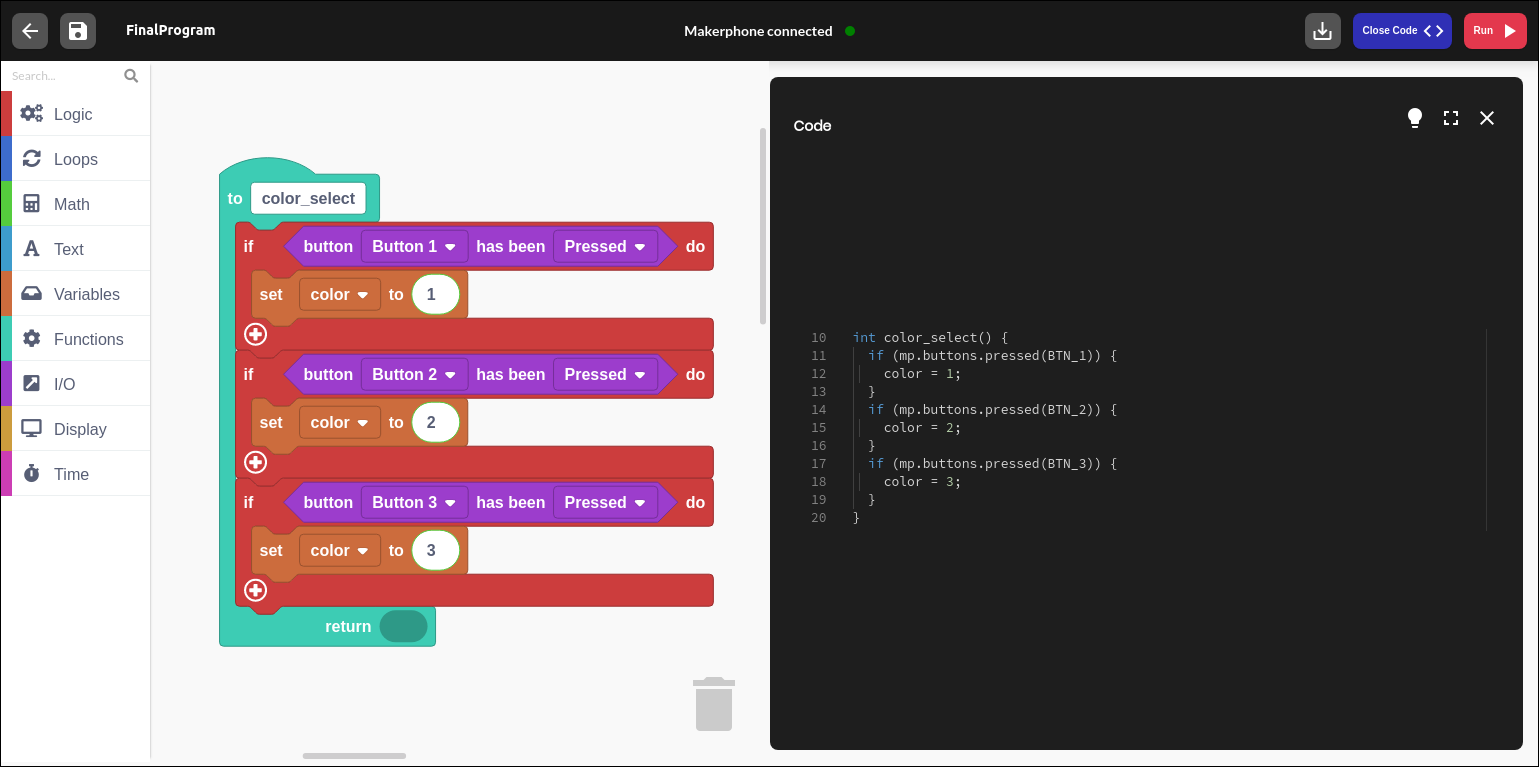
This is probably the easiest way to do it, but it can also be done in other ways.
Pressing on of buttons 1, 2 or 3, this variable changes. We will use it in another function.
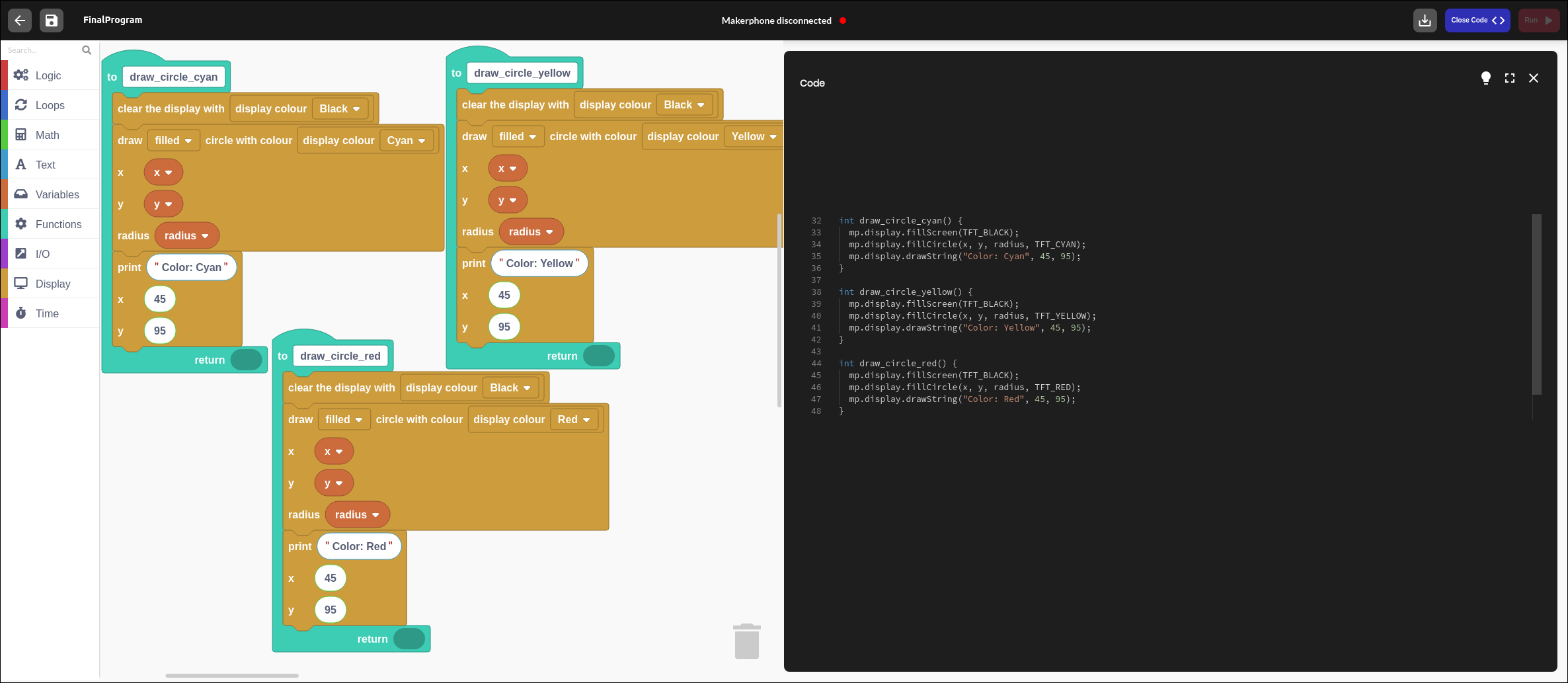
Depending on the color variable that we've previously changed, we are entering one of the three functions.
They all work in the same way but have a tiny little difference that makes them special.
Depending on which function is called, the color of the circle will be different.
Clearing the screen with the black color allows the circle to move swiftly and look like an animation.
Also, the function prints out the color of the circle at the bottom of the screen.
We've already set the font type and font size at the beginning of the program so there is no need in doing that now.
Print data
And finally, we're printing out some additional data on the screen.
Also, the coordinates of the circle center will be shown in the upper left corner.
This will perfectly track the circle along with its size.
Since this function was called after the draw_circle function, this text will always be visible even when the circle is at the same location on the screen.
If you want it to be otherwise, just call the draw_circle function after this one.
The next picture represents the entire program.
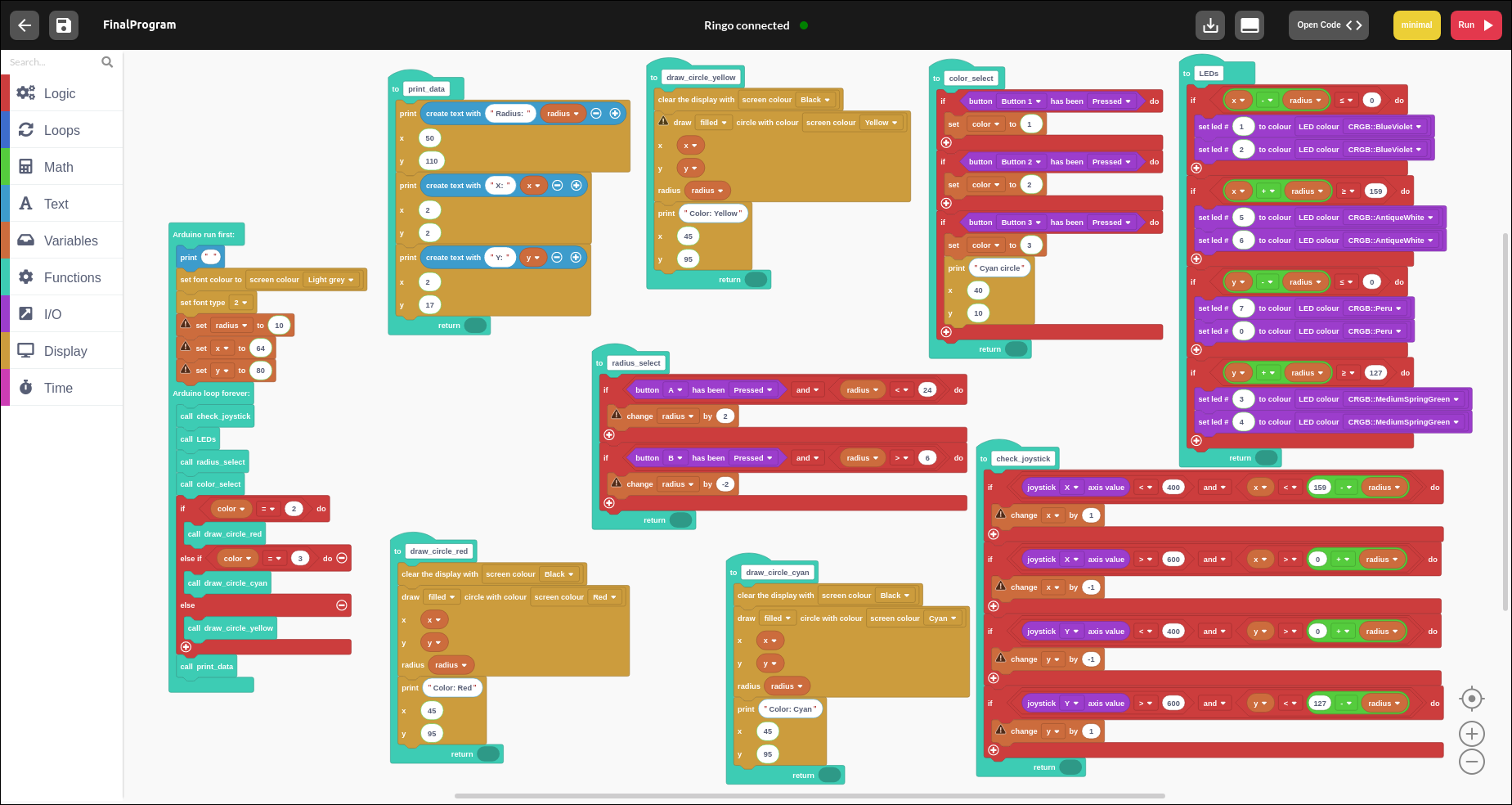
Conclusion
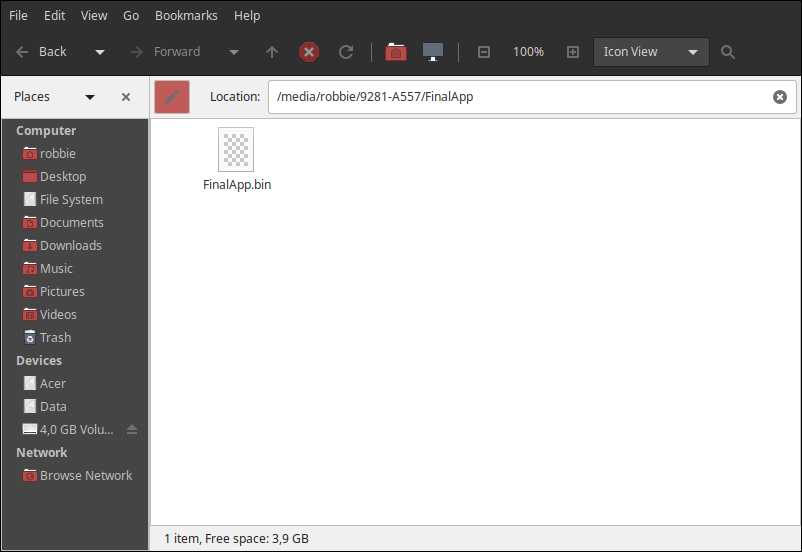
In chapter 3 there is a detailed tutorial on how to do this, so go check it out!
There is a new app on your main menu ready to go!